“Happiness in this world, when it comes, comes incidentally. Make it the object of pursuit, and it leads us a wild-goose chase, and is never attained. Follow some other object, and very possibly we may find that we have caught happiness without dreaming of it.”
― Nathaniel Hawthorne
In this free tutorial by @MammothCompany, learn how to enable your game camera to follow your player in Unity!
Tweet
In this tutorial:
- Part 1: Setup
- Part 2: Writing the Camera Logic
- Part 3: Testing the Script
- Part 4: Offset the Player’s Position
- Part 5: Changing Position Indirectly
- Part 6: Make Movement Smooth
- Part 7: Changing Speed of Camera Follow
You can’t have a game where the player disappears and we never see ourselves again! It’s time to learn how to follow a player with the game camera.
Read on to learn how to make the user to feel like they are running alongside the player.
Part 1: Setup
To follow along with this tutorial, you’re going to need a Unity game open with a player and a velocity. If you don’t have this yet, use our previous tutorial to set up exactly that!
Part 2: Writing the Camera Logic
Making the camera follow the player requires specific logic. Therefore, we will need to make a script for the behavior. Right-click in the Scripts folder, and create a new C# script. Name the script “CameraFollow”.
When the compilation completes, select Main Camera. Drag and drop CameraFollow to a blank space in the Inspector. Double-click on the script to open it.
What will the logic be? We want the camera to lock into a certain position. The position will be the position of the player.
In the CameraFollow class, create a GameObject variable named target. This variable will hold the object that the camera will follow.
public GameObject target;
In the Update method, access the Position property of the Transform component. With the following format, set the property to a new Vector3.
void Update () {
transform.position = new Vector3 ();
}
Within the parentheses, you can set the values of the Vector3.
With the following code, set the camera’s X position to be the same as the target game object’s X position. Set the camera’s Y position to be the same as target’s Y position. Thus when the player moves side to side or up and down, the camera will as well.
void Update () {
transform.position = new Vector3 (target.transform.position.x, target.transform.position.y);
}
So far we have 2 values for the Vector3, but Vector3 is a 3-dimensional direction. We’re missing the position in the Z axis.
Use the following code to set the camera’s Z position to remain the same.
void Update () {
transform.position = new Vector3 (target.transform.position.x, target.transform.position.y, transform.position.z);
}
Note that writing transform.position.z is the same as typing this.transform.position.z. Both ways of writing the code reference the CameraFollow class.
Part 3: Testing the Script
Save the script, and open Unity. When the compilation completes, the Camera Follow (Script) component in the Inspector will have a new property: Target.
Drag and drop Main Camera from the Hierarchy to the Target field in the Inspector. Press Play. The camera will follow the player. Press Stop.
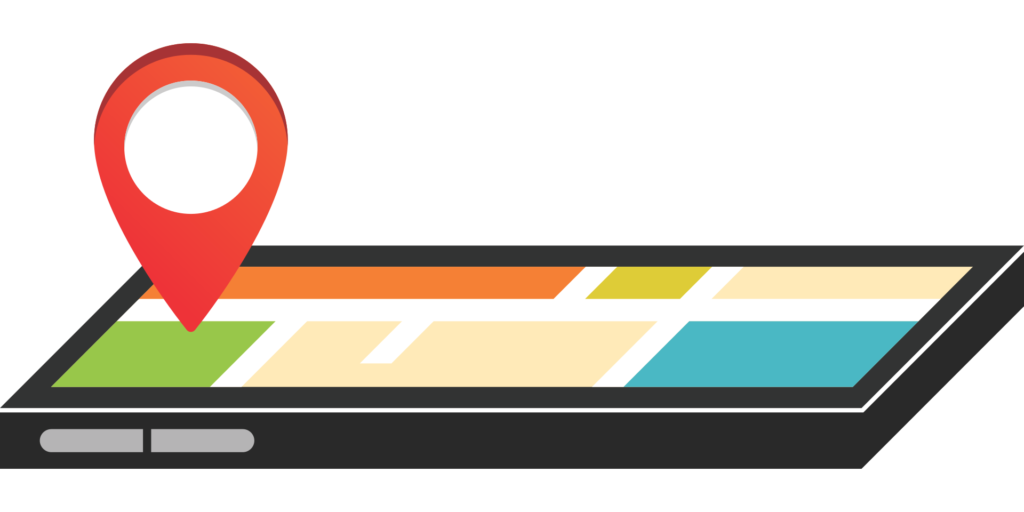
Part 4: Offset the Player’s Position
Currently the player is in the center of the screen. However, because the player is moving to the right, we can have the camera be offset a bit to the right so that the user can see more of what is coming up in the level. We can also look at the floor from higher up.
Open CameraFollow.cs. At the top of the class, create a Vector3 variable named offset.
public Vector3 offset;
In the Update method, add offset.x to the camera’s X position. Add offset.y to the camera’s Y position.
void Update () {
transform.position = new Vector3 (target.transform.position.x + offset.x, target.transform.position.y + offset.y, transform.position.z);
}
Save the file, and open Unity. Change the X value of the Offset property in the Inspector to 2. Change the Y value also to 2. Note that this property has X, Y, and Z fields because we created the offset variable as a Vector3.
Part 5: Changing Position Indirectly
Open CameraFollow.cs. Instead of directly changing the camera’s position by calling transform.position, we can instead make a new vector to contain the camera’s target position.
void Update () {
Vector3 targetPosition = new Vector3 (target.transform.position.x + offset.x, target.transform.position.y + offset.y, transform.position.z);
}
As such, Main Camera’s position will be stored in targetPosition.
Part 6: Make Movement Smooth
To make the camera move smoothly, we can call the Lerp method on the camera’s Position property. The Lerp method is a linear interpolation. It will make a smooth movement from one position to another. Use the following code to set up the Lerp method.
void Update () {
Vector3 targetPosition = new Vector3 (target.transform.position.x + offset.x, target.transform.position.y + offset.y, transform.position.z);
transform.position = Lerp();
}
Lerp’s first parameter will be the position of the camera. You can call this parameter with transform.position.
The second parameter is the position to where we want Main Camera to move. We stored this position in targetPosition. The third parameter is Time.deltaTime to scale the movement speed so that the speed is consistent across different processors.
transform.position = Lerp(transform.position, targetPosition, Time.deltaTime);
Save the script, and open Unity. Press Play. The camera will follow the player more smoothly than before. The camera will also be slightly ahead of the player. Press Stop.
Part 7: Changing Speed of Camera Follow
We can change how quickly the camera follows the player. Open CameraFollow.cs. At the top of the class, declare a float variable named speed. Give it the initial value 5f.
public float speed = 5f;
In the Update method, add speed to the third parameter in the Lerp method like so:
transform.position = Lerp(transform.position, targetPosition, speed * Time.deltaTime);
Save the script, and open Unity. Press Play. The camera will follow the player faster than before.
You may notice that the cube does not move perfectly smoothly. This occurs because Player moves differently from the camera because Player uses Rigidbody.
Press Stop. To fix this problem, open CameraFollow.cs. Rename Update “FixedUpdate”. Thus the method will be called in a physics way. The code in the method will be updated along with Player’s physics.
Review
- Part 1: Setup
- Part 2: Writing the Camera Logic
- Part 3: Testing the Script
- Part 4: Offset the Player’s Position
- Part 5: Changing Position Indirectly
- Part 6: Make Movement Smooth
- Part 7: Changing Speed of Camera Follow
—Team Mammoth from Mammoth Interactive INC. Tutorial by Glauco Pires and Transcribing by Alexandra Kropova